Code Refactoring: Meaning, Benefits and Techniques
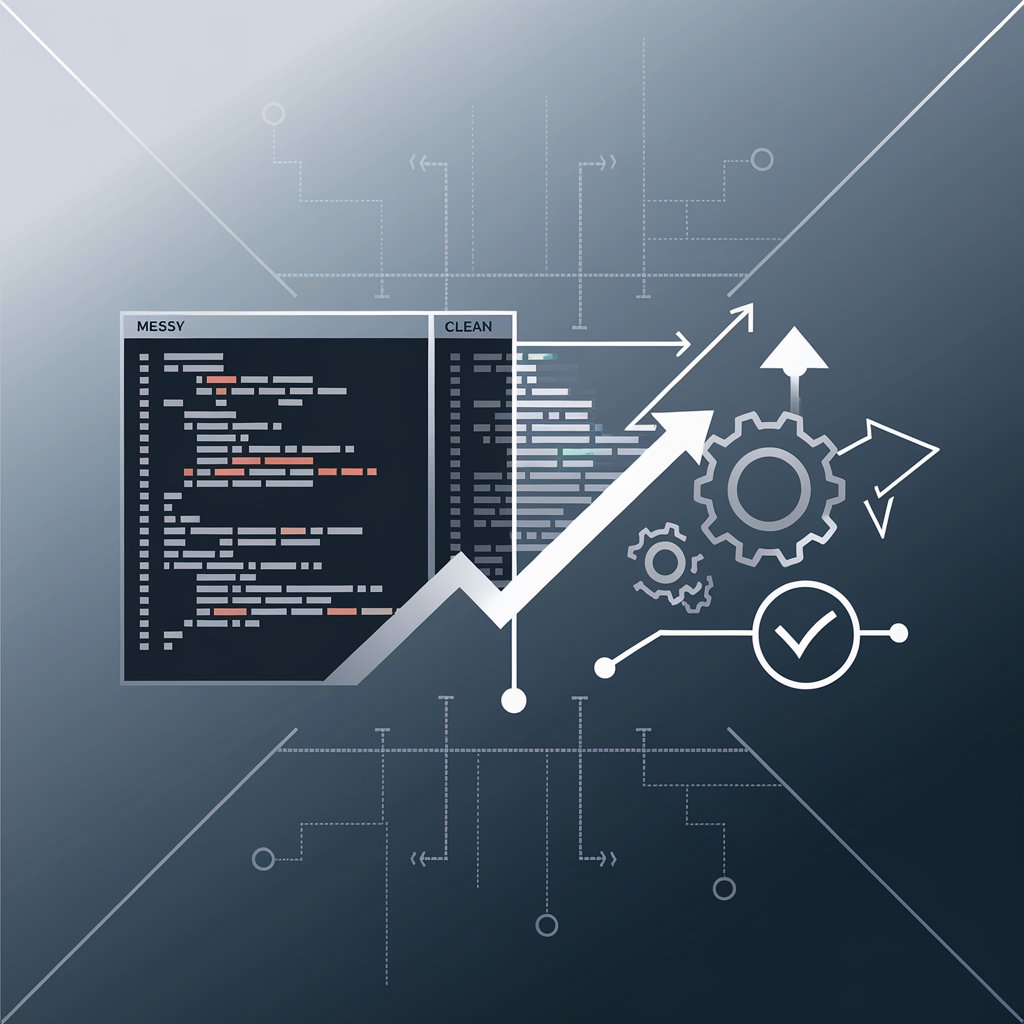
As a software developer, you’re likely familiar with the concept of code refactoring. This essential practice involves restructuring existing code without changing its external behavior. By refactoring, you can improve your codebase’s readability, maintainability, and efficiency. However, many developers struggle to implement effective refactoring techniques or fully grasp their benefits.
In this article, you’ll explore the meaning of code refactoring, understand its numerous advantages, and learn practical techniques to enhance your development process. Whether you’re a seasoned programmer or just starting your coding journey, mastering the art of refactoring will elevate your skills and contribute to more robust, scalable software solutions.
What is Code Refactoring?
Code refactoring is the process of restructuring existing computer code without changing its external behavior. It’s like renovating a house, you’re improving its internal structure and efficiency without altering its outward appearance. The goal is to improve the code’s readability, reduce complexity, and enhance maintainability.
Refactoring involves several techniques, such as simplifying methods, eliminating redundancies, and improving code organization. It’s not about fixing bugs or adding features, but rather about cleaning up the codebase to make it more efficient and easier to work with in the future.
Remember, refactoring is an ongoing process. It’s about continuously improving your code to maintain its quality and extensibility over time.
Why Refactor Code? The Benefits of Code Refactoring
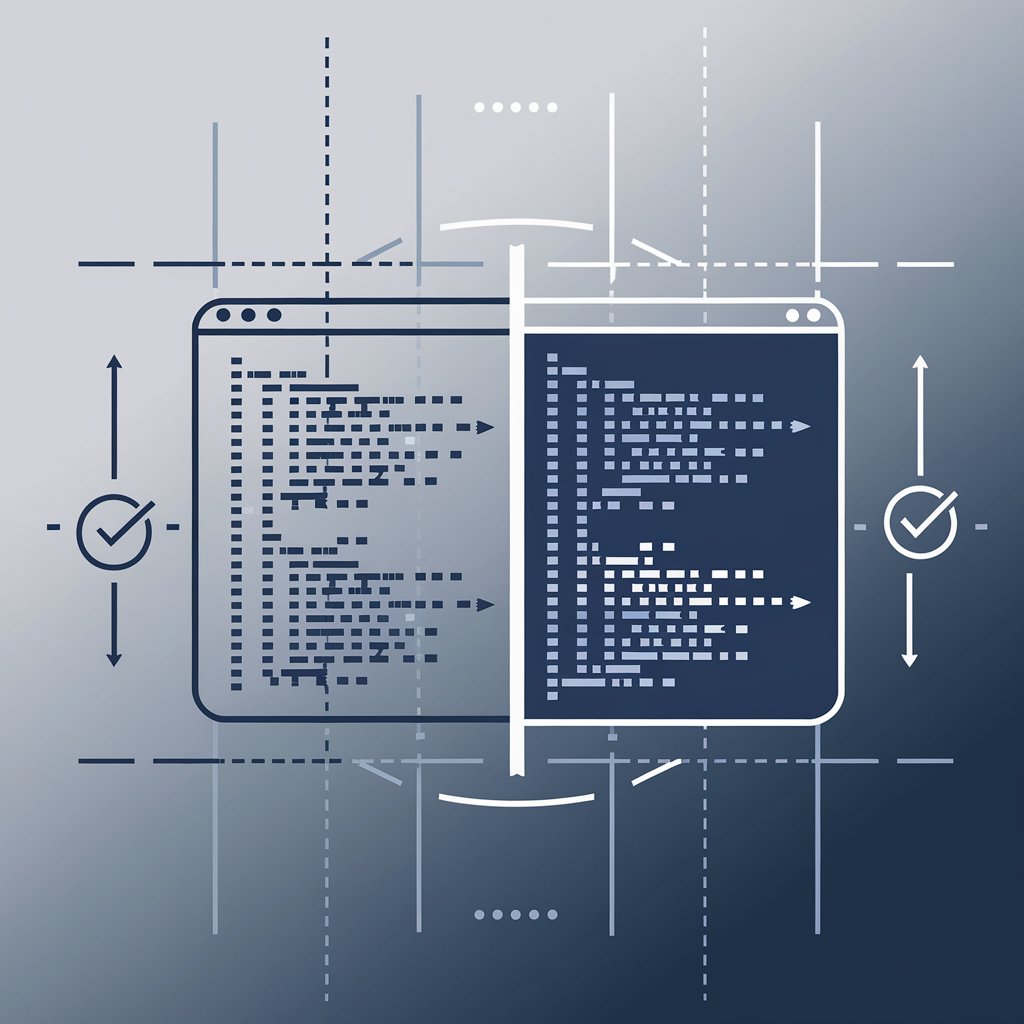
These are some of the benefits of code refactoring:
Improved Code Readability and Maintainability
Refactoring enhances code clarity, making it easier for developers to understand and maintain. By simplifying complex structures and removing redundancies, you’ll create more intuitive code that’s less prone to errors.
Enhanced Performance and Efficiency
Through code refactoring, you can optimize algorithms and streamline processes, resulting in faster execution times and reduced resource consumption. This leads to improved overall system performance.
Better Scalability
Refactored code is typically more modular and flexible, allowing for easier expansion and adaptation to new requirements. This scalability is crucial for long-term project success and growth.
Reduced Technical Debt
By addressing code issues early and consistently, you minimize the accumulation of technical debt. This proactive approach saves time and resources in the long run, preventing major overhauls later.
Easier Bug Detection and Fixing
Cleaner, refactored code makes it simpler to identify and resolve bugs. With improved structure and organization, you can pinpoint issues more quickly and implement fixes more effectively.
Improved Collaboration
Well-refactored code facilitates better teamwork. When your codebase is clean and well-organized, it’s easier for multiple developers to work on the same project simultaneously, enhancing productivity.
Cost-Effective Maintenance
While initial refactoring may require an investment of time, it significantly reduces long-term maintenance costs. You’ll spend less time debugging and more time on innovative features.
When Should You Refactor Code?
Code refactoring is a crucial practice, but timing is everything. You should consider refactoring when your codebase exhibits signs of technical debt. This occurs when quick fixes and shortcuts accumulate, making the code harder to maintain and expand.
Key Indicators for Refactoring
Refactoring becomes necessary when you notice:
- Duplicated code: Multiple sections with similar functionality
- Complex methods: Functions that are overly long or difficult to understand
- Feature envy: A class that seems more interested in another class’s data
- Shotgun surgery: When a single change requires multiple small modifications across the codebase
Balancing Act
While refactoring is beneficial, it’s essential to strike a balance. You shouldn’t refactor constantly, as it can slow down development. Instead, aim for regular, targeted refactoring sessions. This approach helps maintain code quality without disrupting your project’s progress or deadlines.
How Do You Refactor Code
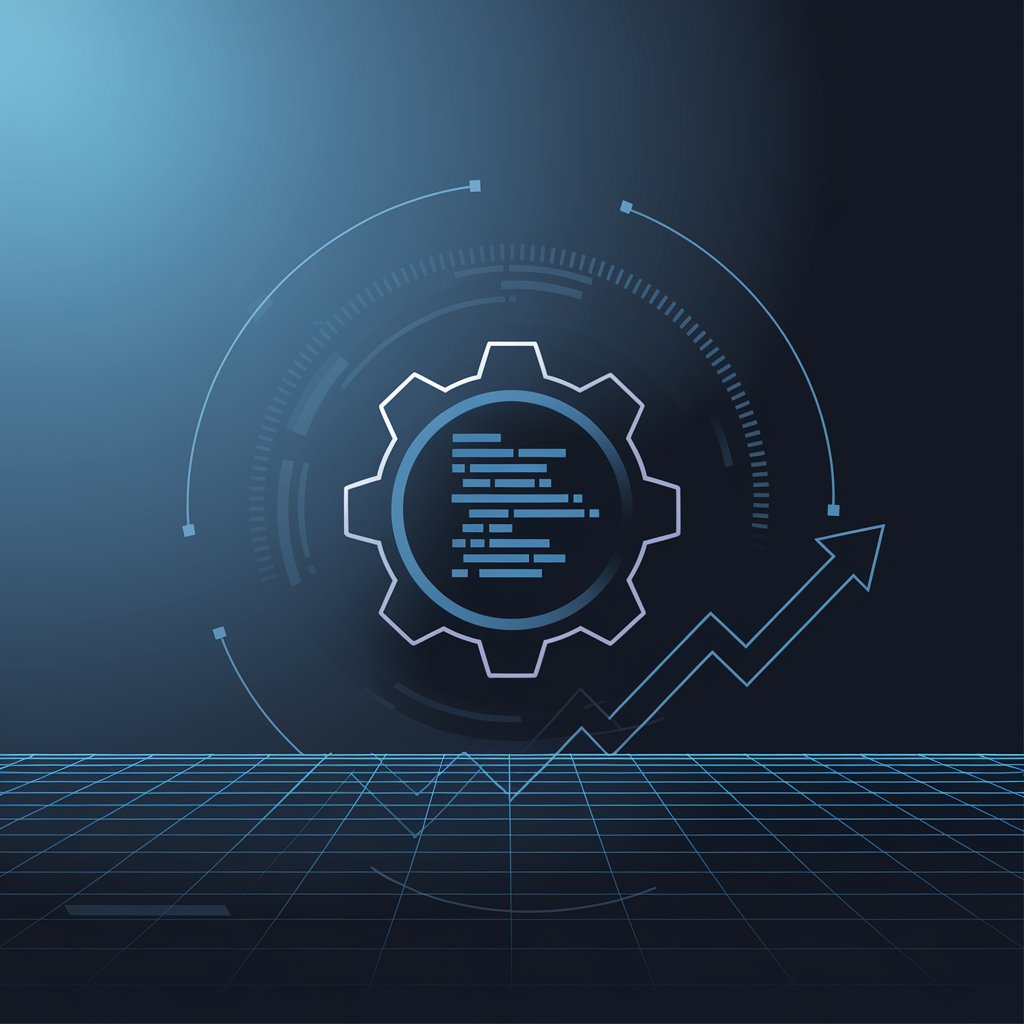
To refactor code follow these steps:
Identify Code Smells
Begin by recognizing code smells, indicators of poor design or implementation. These may include duplicate code, long methods, or complex conditional statements. Use static analysis tools to help detect potential issues.
Apply Refactoring Techniques
Implement proven refactoring methods to address identified problems. Extract methods to break down long functions, use design patterns to improve structure, and eliminate redundancies. Rename variables and functions for clarity, and reorganize code to enhance readability.
Test Continuously
Maintain a comprehensive test suite and run it frequently during the refactoring process. This ensures that changes don’t introduce new bugs or alter existing functionality. Utilize automated testing tools to streamline this crucial step.
Review and Iterate
After implementing changes, review the refactored code with team members. Seek feedback, discuss improvements, and iterate as necessary. Remember, code refactoring is an ongoing process that requires continuous attention and refinement to maintain code quality and efficiency.
Code Refactoring Techniques You Should Know
Extract Method
This technique involves taking a code fragment and turning it into a method. By extracting complex logic into separate methods, you improve readability and reduce duplication. It’s particularly useful when you have long methods or repeated code segments.
Red-Green-Refactor
This technique involves writing a failing test (red), implementing the simplest code to pass the test (green), then refactoring to improve code quality. It ensures functionality while optimizing structure.
Composing Method
Break down complex methods into smaller, more manageable pieces. Extract reusable code segments into separate methods, improving readability and maintainability.
Refactoring by Abstraction
Create abstract classes or interfaces to generalize common functionality. This technique reduces code duplication and enhances flexibility for future changes.
Simplifying Method Calls
Streamline method parameters and return values. Use method object pattern to encapsulate complex parameter lists, making your code more intuitive and easier to understand.
Code Refactoring Best Practices
Keep It Simple
Simplify complex code by breaking it into smaller, more manageable functions. This improves readability and makes future modifications easier. Remember, less is often more when it comes to efficient code.
Follow the DRY Principle
“Don’t Repeat Yourself” is a fundamental refactoring practice. Identify and eliminate code duplication by creating reusable functions or modules. This reduces redundancy and makes your codebase more maintainable.
Use Meaningful Names
Choose clear, descriptive names for variables, functions, and classes. Well-named elements make your code self-explanatory, reducing the need for extensive comments and improving overall comprehension.
Implement Design Patterns
Utilize established design patterns to solve common programming challenges. These tried-and-true solutions can significantly enhance your code’s structure and efficiency.
Conduct Regular Code Reviews
Frequent peer reviews help identify areas for improvement and ensure consistent coding standards. This collaborative approach leads to higher quality code and promotes knowledge sharing within your team.
What is the Difference Between Code Optimization and Code Refactoring?
While both code optimization and code refactoring aim to improve software quality, they serve different purposes. Code optimization focuses on enhancing a program’s performance, speed, and efficiency. It involves tweaking algorithms, reducing memory usage, and minimizing execution time. Developers optimize code to make it run faster or use fewer resources.
On the other hand, code refactoring is about improving the internal structure and design of existing code without changing its external behavior. The goal is to make the code more maintainable, readable, and easier to understand. Refactoring techniques include reorganizing code, eliminating redundancies, and simplifying complex logic.
Key differences:
- Purpose: Optimization improves performance; refactoring enhances code quality
- Focus: Optimization targets efficiency; refactoring targets maintainability
- Outcome: Optimization may alter functionality; refactoring preserves external behavior
Understanding these differences helps developers choose the right approach for their specific needs when working on code improvement projects.
When You Don’t Need Refactoring
While code refactoring is often beneficial, there are situations where it may not be necessary or advisable. You should avoid refactoring when:
The code is already clean and efficient
If your codebase is well-structured, easily maintainable, and performs optimally, there’s no need to refactor. Unnecessary changes could introduce new bugs or complexity.
Time and resources are limited
Refactoring requires time and effort. If you’re working under tight deadlines or with limited resources, it’s better to focus on delivering functional code rather than perfecting existing structures.
The code is slated for replacement
When a system or module is scheduled for complete overhaul or replacement in the near future, investing time in refactoring may not be cost-effective. Instead, focus on maintaining the current functionality until the new version is ready.
Only refactor when you notice code smells, such as duplicate code, long methods, or complex conditional statements. It’s also beneficial before adding new features or after completing a major functionality.
What are the Risks of Refactoring?
While refactoring can improve code quality, it may introduce new bugs if not done carefully. It can also be time-consuming and potentially disrupt development schedules. Always test thoroughly after refactoring to ensure functionality remains intact.
Conclusion
As you embark on your code refactoring journey, remember that it’s an ongoing process, not a one-time event. By consistently applying the techniques discussed, you’ll gradually improve your codebase’s quality, maintainability, and efficiency.
While refactoring may seem time-consuming initially, the long-term benefits far outweigh the short-term costs. Cleaner, more modular code will save you and your team countless hours in the future, reducing technical debt and making your software more adaptable to change. Embrace refactoring as a fundamental part of your development workflow, and you’ll find yourself creating more robust, scalable, and elegant solutions. Your future self, and your colleagues, will thank you for it.